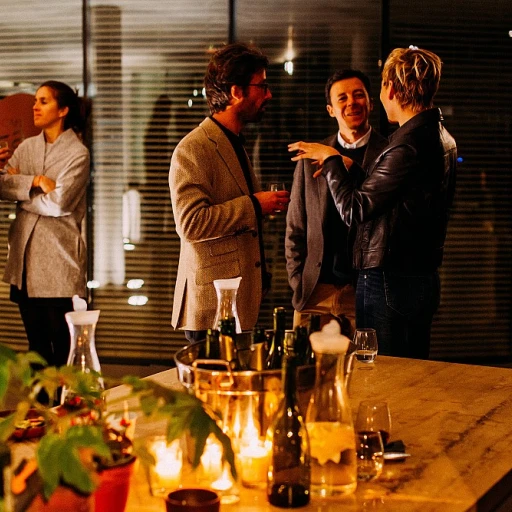
Understanding Verilog's switch case
Getting to Grips with the Verilog Case Construct
When diving into the world of digital design with Verilog, understanding the case construct becomes instrumental. This powerful statement allows developers to simplify the decision-making process in their code by specifying different case items based on the case expression values. This makes the case statement not just a necessity but a tool for efficient and cleaner coding practices.
In its essence, a case statement in Verilog functions similarly to the switch cases found in many other programming languages. The basic structure involves specifying a particular input or variable, known as the sel, followed by multiple potential outputs depending on the value of this input. Here’s how a typical case block is structured in Verilog:
always @(posedge clk) begin
case(sel)
value1: output_reg
In this example, the case expression sel dictates which case statement is executed. If there's no direct match, the default statement takes charge, ensuring a fail-safe mechanism is in place. The use of default is crucial, as it defines a pathway for conditions that aren’t explicitly covered by the other case item entries.
For practitioners aiming to deepen their Verilog expertise, it’s beneficial to review comprehensive resources. Engaging with relevant literature is paramount for mastering such constructs, so exploring options like this list of recommended readings can be invaluable.
Practical Applications of switch case
The Importance of Practical Application in Verilog's Case Statement
To truly understand and master Verilog's switch case, practical application is key. The case statement in Verilog is not just a theoretical concept; it is widely used to control the flow of digital design projects through conditional expressions. In digital systems, conditions vary based on signal inputs and state changes. By using a case statement, specific outputs are matched to given inputs, enhancing the design's efficiency and performance. A practical example of a case statement includes a finite state machine where each state determines the next action based on certain conditions. Here is an example code snippet: verilog always @(posedge clk) begin case(sel) 2'b00: output_reg = item0; 2'b01: output_reg = item1; 2'b10: output_reg = item2; default: output_reg = default_value; endcase end This block of code shows how `sel` inputs can control the data flow and output a specific value depending on the matching condition. The block ensures that only the appropriate statement is executed when the defined conditions are met. The inclusion of a default statement provides a fallback option when none of the given conditions are satisfied, preventing unpredictable behavior. Furthermore, mastering Verilog's switch case involves understanding different variants such as `casez` for don't-care conditions, which can lead to more sophisticated and versatile designs. For example, in scenarios when you deal with signals that have unknown or irrelevant bits, `casez` and `case` syntax allows for more adaptive and robust design solutions. Incorporating these practical applications in digital projects not only sharpens your Verilog skills but fortifies the foundational understanding of digital circuit design as it pertains to statement execution and efficient signal handling. For additional insights, consider exploring the best books for mastering emotional regulation, which can indirectly enhance focus and discipline when learning complex subjects such as Verilog.Common Pitfalls and Challenges
Navigating the Obstacles in Verilog's Switch Case
As with any coding practice, making effective use of Verilog's switch case involves avoiding certain pitfalls and challenges. A fundamental understanding of the case statement is vital, yet even seasoned designers can encounter missteps if not vigilant. Here are some common issues many face when working with Verilog's switch case:
- Unmatched Case Items: One frequent error is an oversight in ensuring that all possible input values are represented within the case statement. Leaving out potential case items might result in unintended default behavior or misinterpretations of what should be executed.
- Neglecting the Default Statement: While the default statement is optional, it's crucial to cover unanticipated input values. Without it, unexpected data sel inputs might lead to ambiguous signal assignments or floating signals.
- Signal Overlaps in Expressions: When specifying case expressions, care must be taken to ensure there's no overlap, particularly in casez or statement case using bxx sel patterns. Overlapping expressions can cause conflicts in determining the correct output reg for a given state.
- Mismatched Output States: It's essential to confirm that every case item leads logically to its corresponding output statements. Failing to do this can disrupt the logical flow, misrepresenting endcase or misaligning with the current state expectations.
Understanding these pitfalls is only a part of the learning journey in digital design. To deepen your understanding of mastering coding challenges, consider exploring resources focused on continuous learning principles that can offer strategic insights and keep you well-prepared for tackling complex scenarios in programming.
Advanced Techniques with switch case
Implementing Advanced Logic with Switch Case
Delving into more sophisticated uses of Verilog's switch case can help enhance the logic and clarity of your code. When you want to efficiently manage a multitude of conditions, Verilog case statements stand as a vital tool, offering streamlined control over your logical flow. By strategically using the case item and case expression, you create a cohesive control structure that directs how statements are executed. One important technique involves the use of the casez expression, allowing you to match patterns using wildcard expressions with 'z' as unknown values. This is particularly useful in handling don't-care conditions in digital design. Similarly, employing the bxx sel can assist in managing selection signals where partial bits are to be neglected. In the scenario of complex state machines, you can utilize nested case statements to handle multiple layers of conditions based on the current state or data paths. For instance, let’s consider a Verilog block where input signals define different stages: verilog always @(posedge clk) begin case (sel) 2'b00: output_reg Continuous Learning in Digital DesignEnhancing Your Digital Design Skills Continually
To truly excel in digital design, one must embrace the principle of continuous learning, especially when mastering Verilog's switch case constructs. This involves a dedication to not just understanding the basics, such as the statement case, default statement, and endcase, but also delving into more intricate aspects like the casez statement and leveraging the full potential of Verilog for complex projects.
Building a strong foundation requires actively seeking out practical experiences and real-world examples. Experimenting with various input and output configurations, as well as understanding how to properly set up case expressions and manage the state accurately, provides the groundwork for tackling more sophisticated problems.
As projects grow in complexity, so does the importance of avoiding common pitfalls. Knowing how to implement the default statement wisely to avoid unexpected behaviors, or ensuring the statement executed aligns with the desired state, can prevent costly design errors.
Continuously updating one's knowledge involves not just practice, but also engaging with the vast amount of academic resources, online tutorials, and expert communities. Engaging in forums and peer discussions allows you to exchange knowledge and gain different perspectives on the application of switch case statements in digital design.
Ultimately, the journey to mastering Verilog is ongoing. While understanding fundamentals is critical, embracing advanced techniques and continually learning from both successes and challenges equips you with the agility needed to adapt to technological advancements in digital design.
Resources for Mastery
Additional Resources for Deeper Understanding
Gaining mastery in managing Verilog's switch case statements necessitates a dive beyond basic knowledge. Whether you're looking to refine your use of the case block or improve your design of state machines, immersing yourself in a variety of resources enhances understanding and application. Consider leveraging the following resources:- Technical Manuals and Documentation: Referencing official documentation and guides provides a foundational understanding of Verilog's syntax and functionalities. These manuals often illustrate how a signal interacts with cases, optimizing for different input values.
- Online Courses and Tutorials: Platforms offering digital design courses can be a valuable asset. These courses often incorporate practical examples showing how case statements transition between states when posedge clk triggers a change or when outputs are determined by input signals.
- Community Discussions and Forums: Engage with communities and forums where continuous learning is prioritized. These platforms allow for sharing insights on overcoming challenges with advanced techniques in Verilog case usage, such as utilizing the casez for pattern matching.
- Code Repositories: Explore public repositories that offer sample code demonstrating effective use of statement verilog structures. Observing projects that effectively handle default statements or employ case item variations can offer new perspectives.
- Webinars and Workshops: Participate in webinars focusing on digital design intricacies. These sessions often delve into complex scenarios, from detailing block executions to managing outputs within specific case expressions.